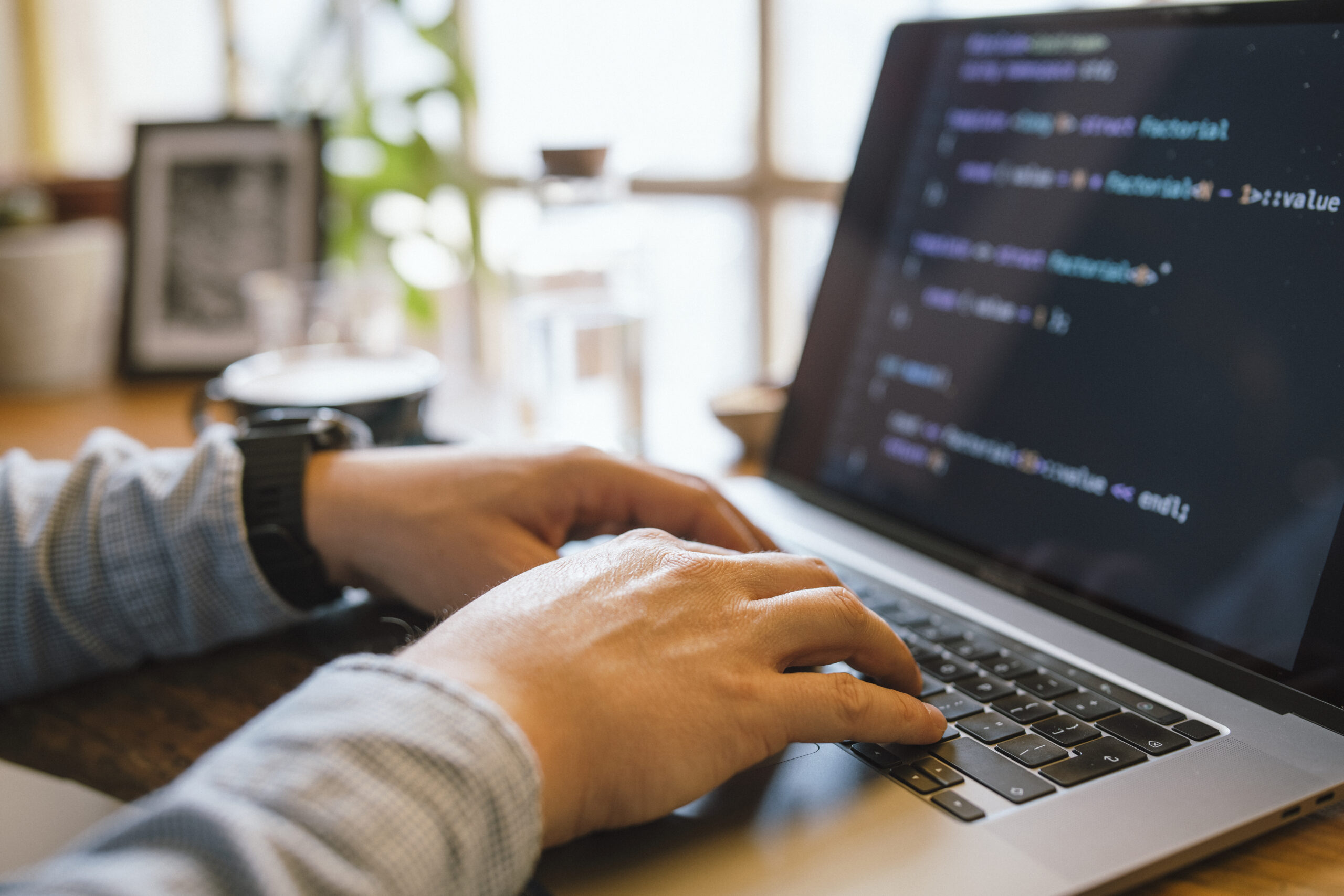
Debugging is Just about the most vital — nonetheless frequently forgotten — competencies within a developer’s toolkit. It's actually not pretty much correcting broken code; it’s about knowing how and why factors go Completely wrong, and Studying to Feel methodically to unravel complications efficiently. No matter whether you are a beginner or perhaps a seasoned developer, sharpening your debugging expertise can help you save several hours of aggravation and drastically boost your productivity. Listed below are numerous procedures that can help builders level up their debugging recreation by me, Gustavo Woltmann.
Master Your Tools
One of the fastest strategies developers can elevate their debugging expertise is by mastering the resources they use each day. Whilst writing code is one Element of enhancement, recognizing ways to communicate with it efficiently in the course of execution is Similarly vital. Modern-day growth environments arrive equipped with impressive debugging abilities — but several developers only scratch the area of what these equipment can do.
Take, such as, an Built-in Improvement Ecosystem (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These resources assist you to set breakpoints, inspect the worth of variables at runtime, phase via code line by line, and perhaps modify code within the fly. When used effectively, they let you notice exactly how your code behaves all through execution, that's a must have for tracking down elusive bugs.
Browser developer instruments, like Chrome DevTools, are indispensable for entrance-finish builders. They allow you to inspect the DOM, keep an eye on community requests, see authentic-time overall performance metrics, and debug JavaScript from the browser. Mastering the console, sources, and network tabs can switch frustrating UI troubles into workable tasks.
For backend or program-amount developers, resources like GDB (GNU Debugger), Valgrind, or LLDB present deep control above functioning processes and memory management. Mastering these tools might have a steeper Finding out curve but pays off when debugging performance concerns, memory leaks, or segmentation faults.
Outside of your IDE or debugger, come to be comfortable with Edition Management devices like Git to understand code background, uncover the precise minute bugs were being introduced, and isolate problematic modifications.
In the end, mastering your applications indicates going past default options and shortcuts — it’s about building an intimate familiarity with your enhancement ecosystem so that when problems arise, you’re not misplaced at midnight. The better you realize your resources, the more time you are able to invest solving the actual trouble rather than fumbling as a result of the procedure.
Reproduce the situation
Among the most important — and sometimes neglected — measures in successful debugging is reproducing the issue. Prior to leaping in the code or earning guesses, builders want to create a consistent ecosystem or circumstance in which the bug reliably appears. Without the need of reproducibility, correcting a bug gets a recreation of chance, normally resulting in wasted time and fragile code variations.
Step one in reproducing an issue is accumulating as much context as possible. Talk to inquiries like: What actions triggered The problem? Which environment was it in — progress, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The greater detail you've, the a lot easier it will become to isolate the exact conditions underneath which the bug occurs.
When you finally’ve gathered adequate information, try to recreate the condition in your local environment. This might suggest inputting the same knowledge, simulating similar consumer interactions, or mimicking procedure states. If The problem seems intermittently, think about producing automated assessments that replicate the edge scenarios or state transitions involved. These assessments not only support expose the condition but additionally protect against regressions in the future.
At times, The difficulty may be surroundings-precise — it'd occur only on specified functioning systems, browsers, or beneath unique configurations. Applying tools like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating such bugs.
Reproducing the issue isn’t only a phase — it’s a way of thinking. It requires patience, observation, as well as a methodical technique. But when you finally can continuously recreate the bug, you're currently halfway to fixing it. Having a reproducible situation, You need to use your debugging equipment additional proficiently, take a look at probable fixes properly, and converse additional Plainly with the staff or people. It turns an summary grievance into a concrete challenge — and that’s in which developers thrive.
Read and Understand the Mistake Messages
Mistake messages will often be the most worthy clues a developer has when a thing goes Mistaken. As an alternative to viewing them as irritating interruptions, developers should really study to take care of mistake messages as direct communications in the system. They normally inform you what exactly occurred, where it transpired, and often even why it occurred — if you know how to interpret them.
Start out by looking through the message diligently As well as in complete. Lots of builders, especially when less than time strain, glance at the first line and promptly commence making assumptions. But further within the mistake stack or logs could lie the legitimate root lead to. Don’t just copy and paste error messages into search engines like yahoo — read and recognize them initial.
Crack the error down into sections. Is it a syntax error, a runtime exception, or a logic error? Will it stage to a selected file and line quantity? What module or purpose triggered it? These issues can manual your investigation and position you toward the accountable code.
It’s also handy to know the terminology of your programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java usually observe predictable patterns, and Understanding to acknowledge these can significantly accelerate your debugging process.
Some problems are obscure or generic, As well as in These situations, it’s very important to examine the context during which the mistake happened. Check associated log entries, input values, and up to date variations within the codebase.
Don’t forget about compiler or linter warnings both. These normally precede larger concerns and provide hints about probable bugs.
Finally, mistake messages aren't your enemies—they’re your guides. Understanding to interpret them effectively turns chaos into clarity, helping you pinpoint problems more quickly, minimize debugging time, and turn into a far more successful and self-assured developer.
Use Logging Sensibly
Logging is one of the most powerful resources inside a developer’s debugging toolkit. When employed efficiently, it provides actual-time insights into how an application behaves, aiding you realize what’s taking place beneath the hood with no need to pause execution or stage with the code line by line.
A great logging technique starts with understanding what to log and at what degree. Typical logging ranges include DEBUG, Facts, Alert, Mistake, and Deadly. Use DEBUG for comprehensive diagnostic details in the course of improvement, INFO for general situations (like prosperous start out-ups), WARN for possible issues that don’t crack the appliance, ERROR for precise challenges, and Deadly when the procedure can’t continue on.
Keep away from flooding your logs with excessive or irrelevant details. An excessive amount logging can obscure critical messages and slow down your procedure. Center on crucial events, condition adjustments, enter/output values, and important determination points as part of your code.
Format your log messages Evidently and constantly. Consist of context, which include timestamps, request IDs, and performance names, so it’s simpler to trace challenges in distributed systems or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even easier to parse and filter logs programmatically.
For the duration of debugging, logs let you observe how variables evolve, what conditions are fulfilled, and what branches of logic are executed—all without halting This system. They’re especially worthwhile in production environments the place stepping through code isn’t attainable.
On top of that, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about stability and clarity. That has a well-imagined-out logging solution, you'll be able to decrease the time it's going to take to spot troubles, attain deeper visibility into your programs, and Enhance the Over-all maintainability and reliability of the code.
Assume Similar to a Detective
Debugging is not just a specialized undertaking—it is a form of investigation. To efficiently discover and take care of bugs, developers should strategy the method similar to a detective resolving a secret. This mindset assists break down sophisticated difficulties into workable pieces and follow clues logically to uncover the root trigger.
Commence by collecting evidence. Consider the signs of the challenge: mistake messages, incorrect output, or effectiveness difficulties. Identical to a detective surveys against the law scene, obtain just as much applicable information as you can with out jumping to conclusions. Use logs, exam cases, and person experiences to piece alongside one another a transparent photo of what’s taking place.
Up coming, type hypotheses. Question by yourself: What may be leading to this conduct? Have any modifications recently been made into the codebase? Has this challenge transpired just before below similar instances? The target is usually to narrow down possibilities and detect likely culprits.
Then, check your theories systematically. Try to recreate the condition in a very controlled environment. When you suspect a particular function or ingredient, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, request your code questions and Permit the outcomes guide you closer to the reality.
Shell out close awareness to tiny details. Bugs generally conceal during the minimum envisioned areas—similar to a missing semicolon, an off-by-one error, or a race issue. Be thorough and individual, resisting the urge to patch the issue with no fully comprehension it. Temporary fixes may possibly hide the true trouble, only for it to resurface later on.
Last of all, preserve notes on Anything you attempted and figured out. Just as detectives log their investigations, documenting your debugging method can help you save time for potential difficulties and help Other folks have an understanding of your reasoning.
By pondering just like a detective, builders can sharpen their analytical skills, strategy challenges methodically, and become more effective at uncovering hidden difficulties in complex methods.
Publish Checks
Writing tests is one of the best solutions to help your debugging skills and All round growth performance. Checks don't just help catch bugs early but additionally serve as a safety Internet that provides you self confidence when building improvements towards your codebase. A nicely-tested application is easier to debug because it enables you to pinpoint specifically in which and when a challenge happens.
Begin with unit exams, which give attention to personal features or modules. These tiny, isolated tests can rapidly reveal whether or not a specific bit of logic is Doing the job as envisioned. Every time a examination fails, you quickly know in which to search, considerably decreasing some time used debugging. Device exams are Particularly useful for catching regression bugs—challenges that reappear immediately after Earlier getting fixed.
Future, combine integration exams and end-to-close assessments into your workflow. These aid make sure that many portions of your application do the job jointly easily. They’re particularly handy for catching bugs that take place in complex devices with many elements or services interacting. If a thing breaks, your exams can show you which Portion of the pipeline unsuccessful and beneath what circumstances.
Crafting exams also forces you to definitely Believe critically regarding your code. To test a element effectively, you would like to grasp its inputs, expected outputs, and edge situations. This level of comprehension Normally sales opportunities to better code construction and much less bugs.
When debugging an issue, producing a failing test that reproduces the bug could be a robust first step. After the exam fails regularly, it is possible to focus on repairing the bug and enjoy your check move when The difficulty is solved. This approach makes sure that the exact same bug doesn’t return in the future.
In brief, producing checks turns debugging from the irritating guessing match right into a structured and predictable method—supporting you capture more bugs, more quickly plus much more reliably.
Choose Breaks
When debugging a tricky problem, it’s effortless to be immersed in the situation—staring at your screen for hours, striving Resolution just after Alternative. But Probably the most underrated debugging resources is just stepping absent. Getting breaks can help you reset your intellect, cut down irritation, and infrequently see The difficulty from the new standpoint.
If you're far too near the code for far too very long, cognitive tiredness sets in. You would possibly start out overlooking evident problems or misreading code that you just wrote just hrs earlier. Within this state, your Mind results in being a lot less successful at dilemma-fixing. A short wander, a espresso split, or perhaps switching to a different task for ten–quarter-hour can refresh your target. Several developers report getting the foundation of a difficulty after they've taken time to disconnect, permitting their subconscious operate inside the background.
Breaks also assistance protect against burnout, Specially throughout longer debugging classes. Sitting before a display screen, mentally stuck, is don't just unproductive and also draining. Stepping away helps you to return with renewed Strength as well as a clearer mindset. You would possibly abruptly notice a lacking semicolon, a logic flaw, or perhaps a misplaced variable that eluded you in advance of.
Should you’re trapped, an excellent rule of thumb is usually to set a timer—debug actively for 45–sixty minutes, then take a five–10 moment crack. Use that time to maneuver about, extend, or do some thing unrelated to code. It could really feel counterintuitive, In particular under restricted deadlines, but it in fact leads to more rapidly and more practical debugging Over time.
To put it briefly, using breaks will not be an indication of weakness—it’s a sensible strategy. It provides your Mind space to breathe, enhances your point of view, and helps you stay away from the tunnel eyesight That always blocks your progress. Debugging is actually a psychological puzzle, and relaxation is part of solving it.
Understand From Each individual Bug
Each and every bug you face is a lot more than just a temporary setback—It really is a possibility to mature as being a developer. No matter whether it’s a syntax mistake, a logic flaw, or simply a deep architectural issue, each one can educate you anything important if you make an effort to mirror and examine what went Erroneous.
Get started by inquiring yourself a couple of crucial inquiries when the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with improved practices like device tests, code assessments, or logging? The responses normally expose blind places as part of your workflow or knowledge and assist you Establish stronger coding habits moving ahead.
Documenting bugs will also be a wonderful pattern. Retain a developer journal or retain a log in which you Notice down bugs you’ve encountered, how you solved them, and Everything you discovered. Over time, you’ll begin to see designs—recurring troubles or frequent errors—that you can proactively avoid.
In workforce environments, sharing That which you've uncovered from a bug with your friends could be Particularly powerful. Irrespective of whether it’s by way of a Slack message, a brief publish-up, or a quick awareness-sharing session, serving to Other individuals avoid the similar concern boosts team performance and cultivates a more powerful learning lifestyle.
Much more importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll commence appreciating them as critical areas of your development journey. In spite of everything, a number of the most effective developers are usually not the check here ones who generate excellent code, but individuals that continually master from their blunders.
Eventually, Each and every bug you deal with adds a whole new layer to your ability established. So next time you squash a bug, take a minute to replicate—you’ll arrive absent a smarter, extra capable developer on account of it.
Summary
Enhancing your debugging techniques takes time, follow, and endurance — but the payoff is huge. It can make you a far more efficient, assured, and able developer. Another time you're knee-deep within a mysterious bug, recall: debugging isn’t a chore — it’s an opportunity to become far better at That which you do.